1. 간단한 인터프리터 예제
2. 간단한 실제 사용 예제
1. 간단한 인터프리터 예제
1.1 현재 위치 주소
1.2 최소 최대 주소
1.1 here() : 현재 위치의 주소를 리턴한다.(현재 커서에 있는 위치)
hex(here())
here()
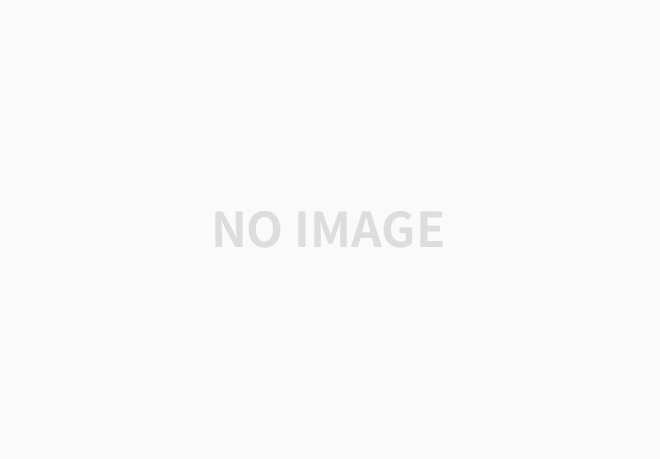
1.2 idc.get_inf_attr(idc.INF_최대,최소_EA) : IDA 데이터 베이스에서 바이너리의 전체 범위에 대한 최대와 최소 주소값 반환
idc.get_inf_attr(idc.INF_MIN_EA)
idc.get_inf_attr(idc.INF_MAX_EA)
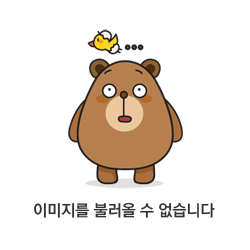
2. 간단한 실제 사용 예제
2.1 세그먼트별 주소 출력 예제
2.2 함수 목록 출력 예제
2.3 전체 명령어 추출 스크립트 예제
2.4 전체 주석 추출 스크립트 예제
2.5 IP 주소와 도메인 이름 추출 예제
2.1 세그먼트별 주소 출력 예제
# 세그먼트의 개수와 세부 정보를 출력
for n in range(idaapi.get_segm_qty()):
seg = idaapi.getnseg(n) # n번째 세그먼트 정보를 가져옴
if seg:
# 세그먼트 이름, 시작 주소, 끝 주소를 가져와서 출력
segname = idc.get_segm_name(seg.start_ea)
start_address = seg.start_ea
end_address = seg.end_ea
print(f"Segment Name: {segname}, Start: {hex(start_address)}, End:{hex(end_address)}")
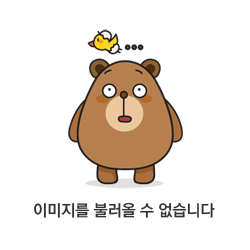
2.2 함수 목록 출력 예제
import idautils
def print_functions():
# 모든 함수들을 순회
for func in idautils.Functions():
# 함수의 시작 주소를 가져옴
start_address = func
# 함수의 이름을 가져옴
func_name = ida_funcs.get_func_name(start_address)
# 함수의 시작 주소와 이름을 출력
print(f"Function Name: {func_name}, Start Address: {hex(start_address)}")
if __name__ == "__main__":
print_functions()
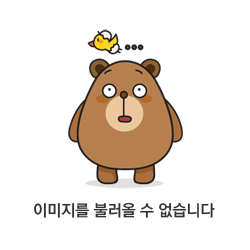
2.3 전체 명령어 추출 스크립트 예제
import idautils
import idc
def find_instructions_with_comments(keyword):
"""
전체 바이너리에서 특정 명령어를 포함하는 코드를 찾아 그 코드의 주소, 명령어, 주석을 출력합니다.
:param keyword: 검색할 명령어 키워드
"""
print(f"Searching for instructions containing '{keyword}':")
print("-" * 50)
for seg in idautils.Segments():
for head in idautils.Heads(idc.get_segm_start(seg), idc.get_segm_end(seg)):
disasm = idc.GetDisasm(head)
if keyword in disasm:
# 명령어에 키워드가 포함되어 있는 경우
comment = idc.get_cmt(head, 0) # 일반 주석
rcomment = idc.get_cmt(head, 1) # 반복 주석
print(f"Address: {hex(head)} - Instruction: {disasm}")
if comment:
print(f" Comment: {comment}")
if rcomment:
print(f" Repeatable Comment: {rcomment}")
print("-" * 40)
if __name__ == "__main__":
# 예를 들어, 'call' 명령어를 포함하는 코드를 찾으려면
find_instructions_with_comments("call")
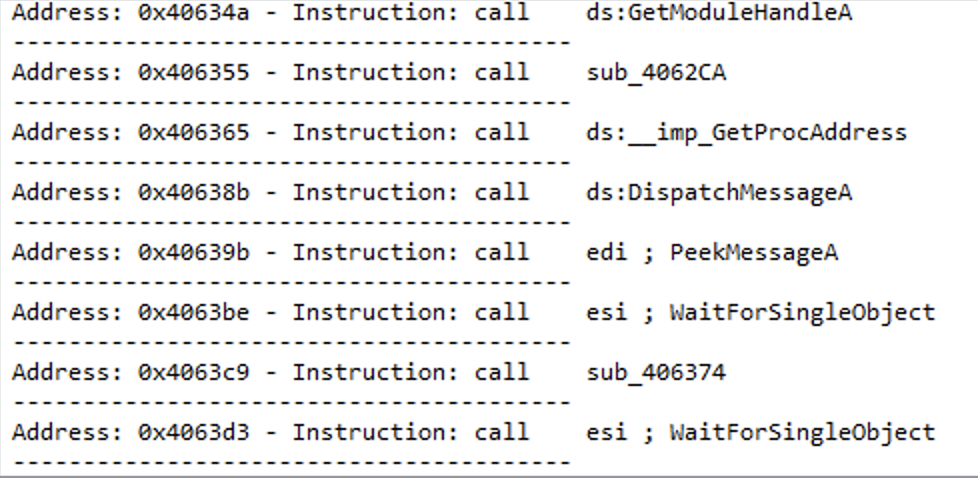
2.4 전체 주석 추출 스크립트 예제
import idautils
import idc
def extract_all_comments():
print("Extracting all comments from the binary:")
print("-" * 50)
for seg in idautils.Segments():
for head in idautils.Heads(idc.get_segm_start(seg), idc.get_segm_end(seg)):
# 주석 가져오기
comment = idc.get_cmt(head, 0) # 일반 주석
rcomment = idc.get_cmt(head, 1) # 반복 주석
if comment or rcomment:
print(f"Address: {hex(head)}")
if comment:
print(f" Comment: {comment}")
if rcomment:
print(f" Repeatable Comment: {rcomment}")
print("-" * 40)
if __name__ == "__main__":
extract_all_comments()
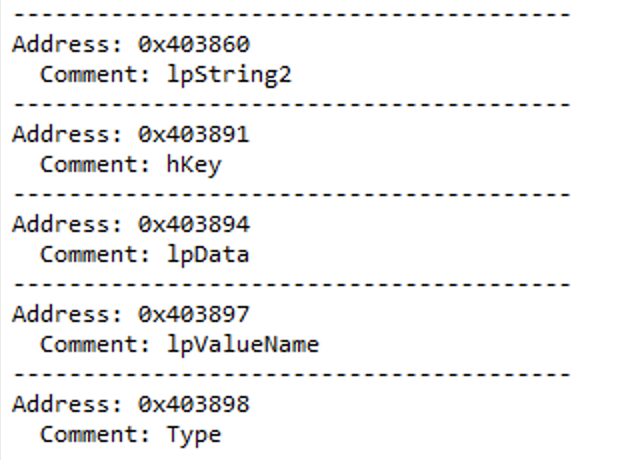
2.5 IP 주소와 도메인 이름 추출 예제
import idautils
import idc
import re
def find_network_iocs():
"""
전체 바이너리에서 네트워크 관련 IOC를 찾아 출력합니다. 이에는 IP 주소와 도메인 이름이 포함됩니다.
"""
ip_pattern = re.compile(r'\b(?:\d{1,3}\.){3}\d{1,3}\b')
domain_pattern = re.compile(r'\b(?:[a-zA-Z0-9-]+\.)+[a-zA-Z]{2,6}\b')
print("Searching for Network IOCs (IP Addresses and Domain Names):")
print("-" * 60)
for seg in idautils.Segments():
for head in idautils.Heads(idc.get_segm_start(seg), idc.get_segm_end(seg)):
disasm = idc.GetDisasm(head)
comments = idc.get_cmt(head, 0) # 일반 주석
rcomments = idc.get_cmt(head, 1) # 반복 주석
strings = idc.get_strlit_contents(head)
search_text = f"{disasm} {comments if comments else ''} {rcomments if rcomments else ''} {strings if strings else ''}"
ip_matches = ip_pattern.findall(search_text)
domain_matches = domain_pattern.findall(search_text)
if ip_matches or domain_matches:
print(f"Address: {hex(head)}")
if ip_matches:
print(f" IP Addresses: {', '.join(ip_matches)}")
if domain_matches:
print(f" Domain Names: {', '.join(domain_matches)}")
print("-" * 60)
if __name__ == "__main__":
find_network_iocs()
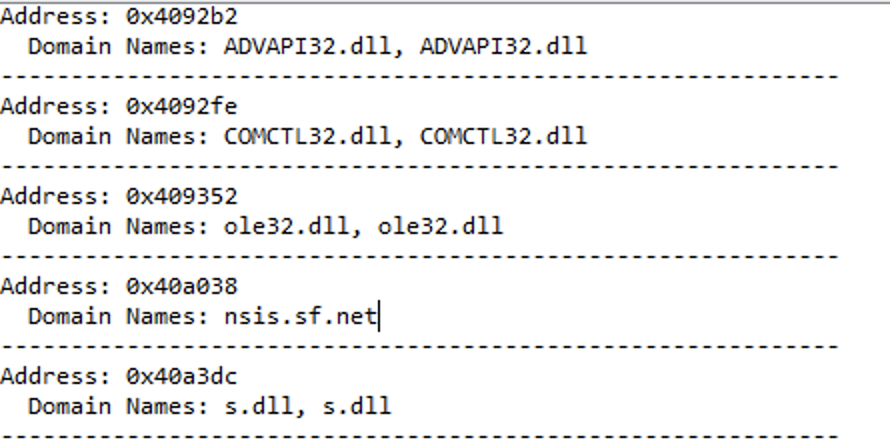
'악성코드 분석' 카테고리의 다른 글
IDA Pro 7.6, Python 3.9, Npcap, scapy 런타임 오류 (0) | 2024.06.05 |
---|---|
IDA Python - socket, whois 모듈 예제 (0) | 2024.05.19 |
IDA Python API 모듈 정리 + 사용법 (python 3.9 ver) (0) | 2024.05.12 |
IDA Pro 7.6 플러그인 설치 방법 - Python3.9(IDA Python) (0) | 2024.05.06 |
Androxgh0st 악성코드와 관련된 알려진 침해 지표 보고서(2024.01.16) -요약 및 정리- (0) | 2024.05.03 |